GetOTP Documentation
Embed Mode
The Embed Mode allows you to perform OTP flow without redirecting to the GetOTP page. OTP form will be shown in a modal.
JS Client
To use Embed Mode in your project, include the script on the page where you want to show the embedded OTP UI.
Once the document is ready, initialize the script. All dependencies will be automatically loaded.
Request for OTP API with Embed Mode
To request for OTP API with Embed Mode, make a POST request similar to an API call for Email OTP / SMS OTP / Voice OTP, but with the following extra parameters
Name | Description | Required | Data Type | Option | Example |
---|---|---|---|---|---|
embed | Type of embed UI | Yes | String | compact | compact |
Examples
Below is an example request for Email OTP with Embed Mode using cURL:
curl -L -X POST 'https://otp.dev/api/verify/' \
-u 'mtbi2w4hlendfpxa1igthcu5p6mzxf7k:mpktanoshzf4c81e3bydjl76ixr9wugv' \
-F 'channel="email"' \
-F 'email="ali@getotp.test"' \
-F 'embed="compact"' \
-F 'callback_url="https://mysite.test/payments/otp-callback/"' \
-F 'success_redirect_url="https://mysite.test/payments/qHgZiJQ8YF/otp-complete/"' \
-F 'fail_redirect_url="https://mysite.test/payments/qHgZiJQ8YF/otp-fail/"'
The response would be a JSON structure, returned with HTTP 200 Code
status code:
{
"otp_id": "kpb9c0a357pdf4jaz05c",
"link": "https://otp.dev/api/ui/verify/kpb9c0a357pdf4jaz05c/email/",
"otp_secret": "dxn07vdzqy7wfblk89r9"
}
Displaying OTP in Modal
Get the link
included in the response and pass it to getotp.showModal()
.
var otp_url = response.link;
// show the otp modal
getotp.showModal(otp_url);
The user will be presented with GetOTP modal form.
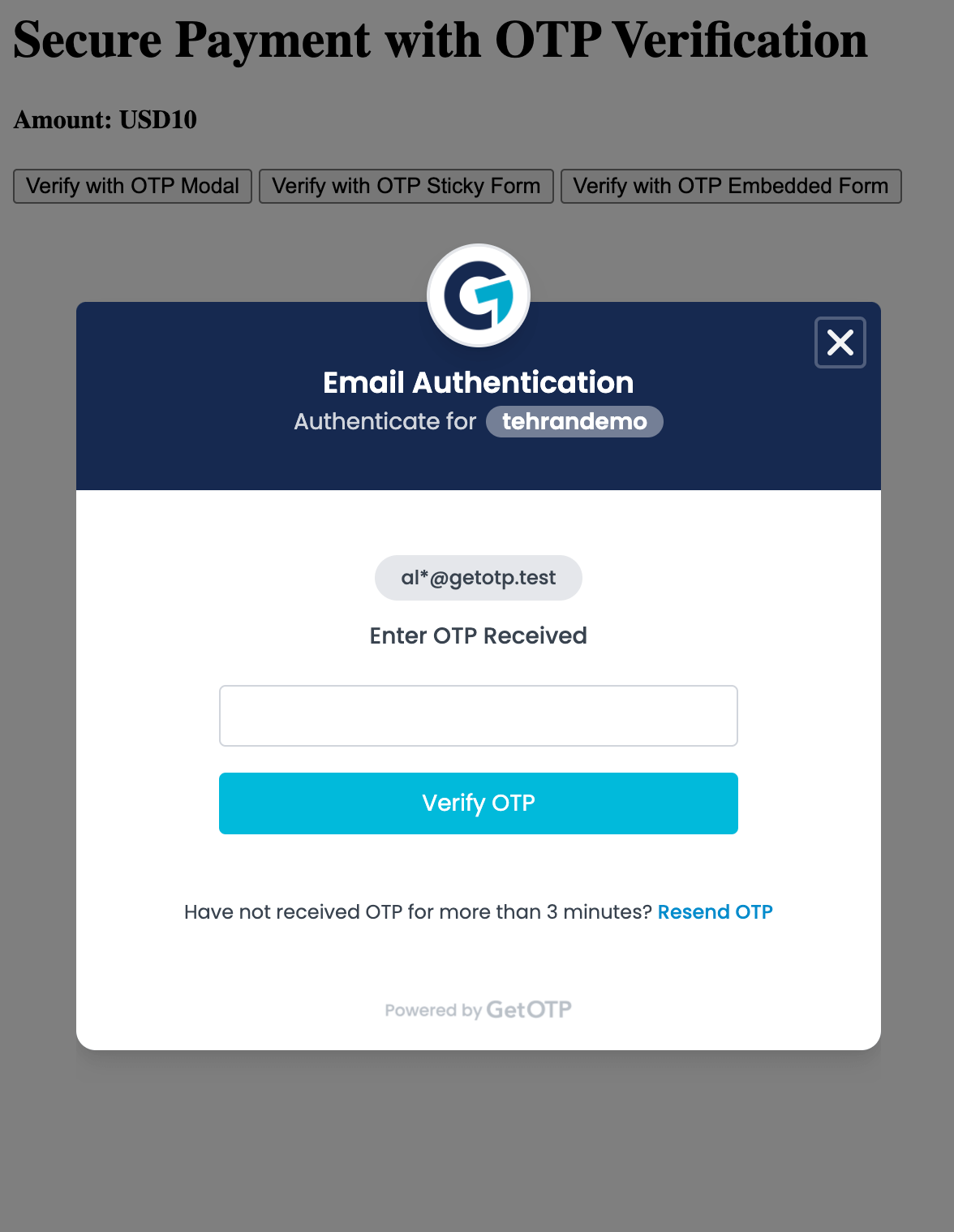
Successful Callback
Once the user fills in the OTP form and submits it, a Javascript Callback will be made. Developer can listen to the onSuccess
event.
getotp.onSuccess(function (payload) {
var callback_otp_id = payload.otp_id;
var redirect_url = payload.redirect_url;
// do something
// getotp.closeModal();
});
Failed Callback
To handle OTP failed event, you need to listen to the onFailed
event.
getotp.onFailed(function (payload) {
var callback_otp_id = payload.otp_id;
var redirect_url = payload.redirect_url;
// do something
// getotp.closeModal();
});
Close Modal
getotp.closeModal();
Reload Modal
If the user reloads the page and you want to show the OTP Modal again.
getotp.reloadModal();
Example with jQuery
Secure Payment with OTP Verification
Available Events
getotp.onOpenModal(function (payload) {
// do something
console.log('after opening otp modal');
});
getotp.onCloseModal(function (payload) {
// do something
console.log('after closing otp modal');
});